A huge amount of existing applications have registration and authentication for users. Maybe every developer in the world has implemented something related to this in their work or while they learned. After the creation of the Django REST framework, Django developers started to implement more and more app-level REST API endpoints.
As a result, an open-source package called dj-rest-auth has been created to provide a set of REST API endpoints to handle user registration and authentication tasks. In this article I will not only talk about this particular package but will also give you some tips to avoid some of the most common problems faced when configuring it.
The dj-rest-auth package
If you are a Django developer and you are coding a REST API with authentication, you will find the dj-rest-auth package very useful. This project is a fork from django-rest-auth that is no longer maintained.
As said, dj-rest-auth provides a set of REST API endpoints to manage user registration and authentication. After an easy installation and configuration, you will have endpoints for:
- User registration with optional activation.
- Login and logout.
- Retrieve and update the Django User model.
- Password change.
- Password reset via e-mail.
- Social media authentication.
You don't need to do too much work to have those functionalities in your app. Besides, given that dj-rest-auth is an open source package, it has the advantage of being used by lots of programmers that can find errors, propose and add improvements, and so on. So, if you use dj-rest-auth you can be sure that you are using code created and maintained by an open source community.
Browsable endpoints
Thanks to the Django REST framework, most of the endpoints that dj-rest-auth provides are browsables. This means that if you have added the package to your Django app, you can test those endpoints through the browser. As an example, if you open the browser and put the URL of the login endpoint, you will see something like this:
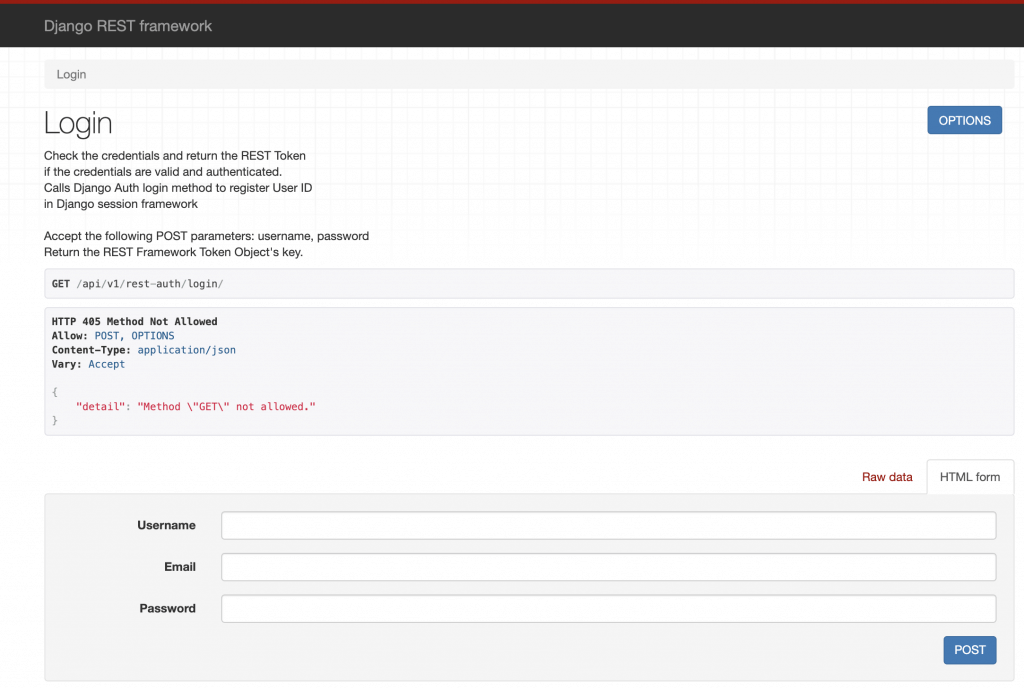
In the image above you can enter a username, email, and password to test the login functionality. The message of Method GET not allowed is not an error. It means that your endpoint doesn't allow the GET method but you can test it doing a POST. That method is possible because the corresponding blue button is shown.
As you can see, it's very useful to very quickly have a nice interface to use while you are coding.
Configuration
The documented installation and minimal configuration of dj-rest-auth is pretty clear and I won't talk about that in this blog post. The main idea of this section is to tell you about the big set of possibilities that you can have in your Django app just by adjusting a couple of parameters. This package uses django-allauth behind so you can take advantage of all the available settings in your own app. For example, you can define these parameters in your app configuration:
- [.c-inline-code]ACCOUNT_EMAIL_REQUIRED[.c-inline-code] ([.c-inline-code]false[.c-inline-code] by default):: If true, the user is required to provide an e-mail address when signing up.
- [.c-inline-code]ACCOUNT_LOGOUT_ON_PASSWORD_CHANGE[.c-inline-code] ([.c-inline-code]false[.c-inline-code] by default): If true, users will automatically be logged out once they have reset their password.
- [.c-inline-code]ACCOUNT_USER_MODEL_EMAIL_FIELD[.c-inline-code] ([.c-inline-code]email[.c-inline-code] by default): The name of the field in the user model containing the email.
- [.c-inline-code]ACCOUNT_USERNAME_REQUIRED[.c-inline-code] ([.c-inline-code]true[.c-inline-code] by default): If true, the user is required to enter a username when signing up.
- [.c-inline-code]ACCOUNT_EMAIL_VERIFICATION[.c-inline-code] ([.c-inline-code]optional[.c-inline-code] by default): Determines the e-mail verification method during signup. The possibilities are one of mandatory, optional, or none.
And many other parameters. As a conclusion, we can say that adjusting a couple of parameters in your Django app settings, you get lots of different behaviors for the registration and authentication.
Note:
To better understand the examples in the next sections:
- You need to follow the dj-rest-auth installation steps.
- Have installed django-allauth.
- Make sure you have the authentication backends in your settings:
CODE: https://gist.github.com/brunomichetti/67521796ad4ee84d220011c1303de52b.js
Customization
If you are coding a REST API with Django and Django REST framework, you may need to customize the functionalities related to the registration and authentication. With those frameworks and dj-rest-auth it's quite simple. You can customize the Django User model, the views, the serializers, the main functionalities, etc.
For example, let's define a custom User model with a unique email, with no username, with a gender and a phone number attribute:
CODE: https://gist.github.com/brunomichetti/1ee6bb40c7f3aecc581782fcc0729bf9.js
In our settings, we have to use the Django parameter called [.c-inline-code]AUTH_USER_MODEL[.c-inline-code] to specify our User model. If the [.c-inline-code]CustomUser[.c-inline-code] model is defined in an app called [.c-inline-code]users[.c-inline-code], we must add:
CODE: https://gist.github.com/brunomichetti/fef0d1d54480a6a7084c9205fae8891a.js
Besides, we have to add in our settings these parameters to use the email as the [.c-inline-code]User[.c-inline-code] identifier in our app:
CODE: https://gist.github.com/brunomichetti/34ee616f057c8b6511c2ffde434847c2.js
Also, let's configure a signup without email verification just for now:
CODE: https://gist.github.com/brunomichetti/2d20efecd239fae59b523420bc60a1d1.js
I'll talk about account verification later.
Now we need to add our custom serializer to define the two added attributes and use them in the registration. The custom register serializer needs to be a child class of the one named [.c-inline-code]RegisterSerializer[.c-inline-code] that dj-rest-auth provides. Also we have to overwrite the save method to set those values that came in the request:
CODE: https://gist.github.com/brunomichetti/3b81921e5d1aab75eb93e6b82100ec4d.js
Now we can't forget to tell dj-rest-auth to use the recently created serializer. Let's add the [.c-inline-code]CustomRegisterSerializer[.c-inline-code] to the settings:
CODE: https://gist.github.com/brunomichetti/c6efcc75edd5efdb418f516a1517073c.js
After this, if we go to the registration endpoint, we would see something like this:
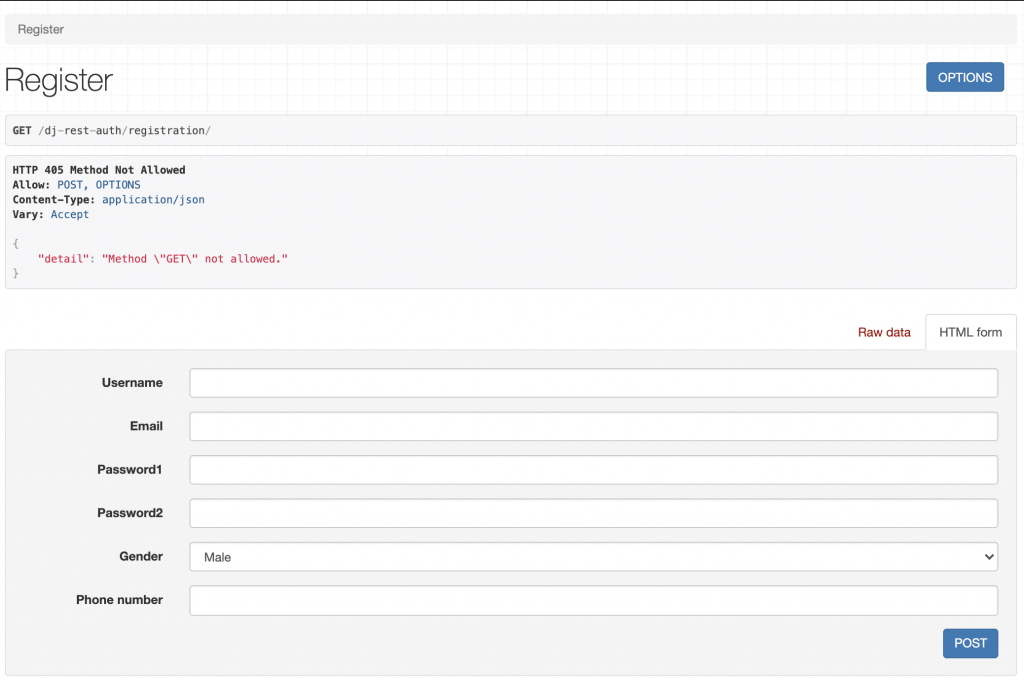
The username appears in the browsable endpoint, but thanks to the configuration you can leave it empty.
And that's it! We have added a customized registration endpoint to our Django app. Now we can create users with email, gender, and phone number, and make some tests around the login functionality too.
Use of JWT
When an app manages authentication, a good and standard implementation of authentication by token authorization is using JWT (JSON web tokens). JWTs are a good way of securely transmitting information between parties because they can be signed, which means you can be sure that the senders are who they say they are. We can easily configure our Django app to use JWT. First we need to install djangorestframework-simplejwt:
Then add to the settings:
CODE: https://gist.github.com/brunomichetti/f9025a6ba6cb0314bd1ee88e971ae37e.js
And we are good to go! We have an app that manages the authentication with JWT.
The User endpoint
If you need to modify or just retrieve the information of an authenticated user; you can use an endpoint provided by dj-rest-auth: [.c-inline-code]dj-rest-auth/user/[.c-inline-code]. It's a very useful endpoint in the case you want the user to be able to modify its own information.
What happens if you have customized the Django user model? Well, in that case you need to overwrite the user serializer to be able to see and update those added attributes. Let's continue with the example of a customized model with a gender and a phone number. In that case we need to tell dj-rest-auth to use the customized serializer already created ([.c-inline-code]CustomRegisterSerializer[.c-inline-code]) as the user's detail serializer, adding this to the settings:
CODE: https://gist.github.com/brunomichetti/d80408028e30156078e83e8363bc66cf.js
With that definition, we can retrieve and update the gender and [.c-inline-code]phone_number[.c-inline-code] of an authenticated user with the named endpoint. Let's say now, that our app only allows the gender to be updated after registration. In the [.c-inline-code]CustomRegisterSerializer[.c-inline-code] the phone number can be modified of course, because we want the user to add that value at the moment of the registration. We can define then another serializer that doesn't allow the phone number to be modified. Let's call it [.c-inline-code]CustomUserDetailSerializer[.c-inline-code] and add it in the same file that is [.c-inline-code]CustomRegisterSerializer[.c-inline-code]:
CODE: https://gist.github.com/brunomichetti/b19356eb513c2938bc20c852dca0d401.js
Then we have to add this as the correct user details serializer in our settings, replacing [.c-inline-code]CustomRegisterSerializer[.c-inline-code] with the new one:
CODE: https://gist.github.com/brunomichetti/9da6e1769b3b28a0c6436010a9019791.js
And this is what you will see in the endpoint of the user:

As you can see in the picture, the only value that can be modified is the gender.
Sign-up with email verification
It's possible to configure email verification at the moment that a user registers. This means that a successfully registered user has to check the inbox and confirm through the received email that he really is the owner of the email account. To do this, go to your settings and turn on email verification:
CODE: https://gist.github.com/brunomichetti/3a0dd172c7e04ad9f362c21057eade80.js
With this, after a correct sign-up your app will send a verification email to the entered account. But you didn't finish: as dj-rest-auth documentation says, after enabling the email verification, you need to add a path to your urls that will be used by the verification view:
CODE: https://gist.github.com/brunomichetti/72169c43edd3fba7e9857e111934c96b.js
If you don't do that, you will get a NoReverseMatch error.
Also, let's use a django-allauth configuration to activate the email account after the user clicks on the link received in the email. Add this to your settings:
CODE: https://gist.github.com/brunomichetti/ba89b2efa129ae7a78b114eddaa1f17a.js
And add this path to your urls file [.c-inline-code]before[.c-inline-code] the definition of the [.c-inline-code]dj-rest-auth[.c-inline-code] registration path:
CODE: https://gist.github.com/brunomichetti/645aefe416a90774e9c7264edd2fecc2.js
That path needs to be there to avoid a template error in dj-rest-auth. As mentioned, dj-rest-auth it's an open source software and sometimes we can find some errors. The good thing of this is that anyone can address the discovered bugs.
Having this configuration, the user will be redirected to the login page after clicking in the received link. Don't forget to set the [.c-inline-code]LOGIN_URL[.c-inline-code] parameter in your settings, otherwise you can get an error if the default value is not a valid URL of your app. During development, you can set the [.c-inline-code]LOGIN_URL[.c-inline-code] as the login endpoint; remember that dj-rest-auth has browsable endpoints thanks to Django REST framework. So you can test sign-up flow by setting for example:
CODE: https://gist.github.com/brunomichetti/7e062d8d1c68ab1e80919d358587d7e9.js
Finally, you need to specify to Django the email backend that is in charge of sending the emails of your app.
Email backend
The Django email backend is the component that handles the sending of an email. You can choose among different possibilities that Django provides. The most common are SMTP and console backend.
You can configure an SMTP server by adding:
CODE: https://gist.github.com/brunomichetti/ad0901e697b5ab77d2773e4dfd278efa.js
You have to choose the [.c-inline-code]EMAIL_HOST[.c-inline-code], for example [.c-inline-code]'smtp.gmail.com'[.c-inline-code]. In the [.c-inline-code]EMAIL_HOST_USER[.c-inline-code] and [.c-inline-code]EMAIL_HOST_PASSWORD[.c-inline-code] parameters put the information of the account that will be the email sender. If you are using Gmail as a mail server you will need to allow less secure apps and display unlock captcha. After this, your Django app will send verification emails for all the new users. I recommend you to use environment variables to keep sensitive information in your settings such as keys, the email host account, and its password, etc.
In real applications the best way to do this is integrating with an email service such as SendGrid.
In the second option, you can locally test the sign-up feature during development, by setting:
CODE: https://gist.github.com/brunomichetti/6b33babfda674beeaa8f1466ae9b50b8.js
In this case the email is sent and received by the console. So after a successful registration you will see in the console something like this:
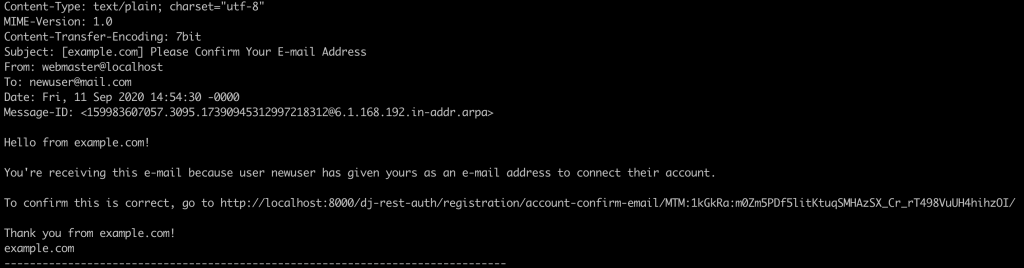
If you open your browser and go to the link shown in the printed email, the user's account will be activated and you will be redirected to the [.c-inline-code]LOGIN_URL[.c-inline-code]. Now you are able to login with the entered email and password.
And there it is! You have configured sign-up with email verification. Let's see how to customize the emails that your application sends.
Email templates
Django allauth provides a few templates that are used by dj-rest-auth at the moment of sending the emails. As shown in previous section, the verification email printed in console has a template defined by the django-allauth package. It's possible to customize those email templates by overwriting a couple of files. First of all, let's create a [.c-inline-code]template[.c-inline-code] folder inside your main Django app folder.
From now on, let's assume that the name of your main Django app folder is [.c-inline-code]myapp[.c-inline-code]; so you need to add this in your [.c-inline-code]TEMPLATES[.c-inline-code] settings:
CODE: https://gist.github.com/brunomichetti/5e68ef062f6185c4689ed5b38599e151.js
Now you have to overwrite the corresponding template files for the desired customization. In this case, the path used by dj-rest-auth will be [.c-inline-code]myapp/templates/account/email/[.c-inline-code]. In there you can add these files to overwrite the ones used by default:
- [.c-inline-code]email_confirmation_message.txt[.c-inline-code]
- [.c-inline-code]email_confirmation_signup_message.txt[.c-inline-code]
- [.c-inline-code]email_confirmation_signup_subject.txt[.c-inline-code]
- [.c-inline-code]email_confirmation_subject.txt[.c-inline-code]
For example, to customize the email confirmation message, you have to create an [.c-inline-code]email_confirmation_message.txt[.c-inline-code] file in [.c-inline-code]myapp/templates/account/email/[.c-inline-code]. You can even reuse some of the content of the default file that you can find in the github repository of django-allauth. Besides, you can set a value to the [.c-inline-code]ACCOUNT_EMAIL_SUBJECT_PREFIX[.c-inline-code] parameter in your settings, to add a prefix to the subjects in the emails of your app.
It's important to say that there are some variables defined in the email templates that are needed and you have to keep them in case you overwrite the files. For example, this is the file of [.c-inline-code]email_confirmation_message.txt[.c-inline-code]:
There you have activate_url, that is the link for the user confirmation. So you can remove for example the site_name if you want (or change it to show a different one) but you definitely have to keep the activate_url value. Otherwise, registered users won't be able to activate their accounts. Summarizing this section, if you decide to overwrite the email_confirmation_message.txt, your project structure will look like this:
Now let's see about the reset password functionality and how to customize it.
Reset password
If you want to have a reset password feature in your app, you can do it very easily with dj-rest-auth. The first thing to say is that by default you can't test it as a browsable endpoint because it will show a [.c-inline-code]NoReverseMatch[.c-inline-code] error. So you need to add this path to your urls file:
CODE: https://gist.github.com/brunomichetti/f5d42170a1f4affe7ca37f42937391f2.js
And now you are good to go! You can test the browsable endpoint which receives the email of the registered user, and dj-rest-auth sends the instructions to reset the password. You need of course to have configured the email backend.
You are able to modify the email template for the reset password functionality too. Assuming that you have already configured the [.c-inline-code]TEMPLATES[.c-inline-code] folder as in the previous section example, you can create a file in [.c-inline-code]myapp/templates/registration/[.c-inline-code] called [.c-inline-code]password_reset_email.html[.c-inline-code] to overwrite the one used by default. Why do you have to define it there? Because is the default path used by Django. You can even reuse some code of the default file, that looks like this:
As said in the email validation section, remember to keep some of the needed variables in the template. In this case the most important is the [.c-inline-code]url[.c-inline-code] with the [.c-inline-code]uid[.c-inline-code] and the [.c-inline-code]token[.c-inline-code] that are required to update the user's password.
After these steps, you have finished the reset password functionality customization! Taking the previous section into account, your project folder would be now like this one:
Let's see in the last section how we can modify some of the default variables used in the templates.
Customize variables used in the templates
Let's think about this example: What if you want to modify the URL sent by default in the reset password email template? Well, Django allows you to define variables to be used in the templates by creating your own template tags. The first thing you need to do is define the custom value desired for the URL in your settings:
CODE: https://gist.github.com/brunomichetti/bda9713a08680e4998415577340ffb2b.js
Then you have to create two files [.c-inline-code]under myapp/templatetags/[.c-inline-code]: One has to be an empty [.c-inline-code]__init__.py[.c-inline-code] file, and the other one registers and gets the tags. Let's call it [.c-inline-code]password_reset_template_load.py[.c-inline-code]:
CODE: https://gist.github.com/brunomichetti/8342e2a779d84483368823e033d4b601.js
Finally, go to your customized email template that you have created to overwrite the default one, and load the defined value by adding [.c-inline-code]{% load password_reset_template_load %}[.c-inline-code] at the top of the file, and after that add the line that has the custom value (in this particular case don't forget the [.c-inline-code]uid[.c-inline-code] and [.c-inline-code]token[.c-inline-code]):
With these settings you have defined your custom template tags. I'm going to show you the final project structure with all the customization that I talked about:
From now on, the email for password reset will show a customized URL. Remember that this was an example, you can create any template tag you want and use it in any template you have.
Notes
- If while trying this you have an error that shows [.c-inline-code]Site matching query does not exist.[.c-inline-code], you can solve it by adding [.c-inline-code]SITE_ID = 1[.c-inline-code] to your settings.
- When you create your own templatetags make sure that the main app is added in the [.c-inline-code]INSTALLED_APPS[.c-inline-code] list in your settings.
Summary
I have presented a very useful package to handle registration and authentication with a set of REST API endpoints. With minimal configuration, you can add endpoints to your Django app that already have those features implemented. We reviewed some advantages of the package, how easy it is to customize it, and code snippet examples.
In addition to this, I have shown you how to implement email validation at sign-up, and how to customize the emails that are sent by your app. We learned about the reset password functionality, and finally how to customize the templates with your own desired values. I recommend using the presented package because it solves lots of common problems and provides a set of features that are very important for most applications. Maybe in the future, you can not only use it but also contribute to it.