Testing in NodeJS, like in any other programming language, is crucial for ensuring the quality, reliability and stability of your code. Here are some of the many reasons why testing is important for your application:
- Bug detection: Testing helps identifying and catch bugs early in the development process. By writing tests for your code, you can verify that it works as expected and catch potential issues before they become critical problems in production.
- Code maintainability: Testing promotes code that is easier to maintain and refactor. When you have a comprehensive test suite, you can make changes to your codebase with more confidence, knowing that you can quickly verify if your changes have introduced any regressions.
- Code documentation: Tests serve as living documentation for your code. They provide examples and use cases that demonstrate how your code should behave, making it easier for other developers (including yourself) to understand and use your codebase.
- Continuous integration and deployment (CI/CD): Testing is an essential part of modern software development practices, including CI/CD pipelines. Automated tests allow you to integrate them into your deployment process, ensuring that only code that passes all tests gets deployed to production, reducing the chances of introducing bugs or breaking existing functionality.
Overall, testing is a crucial practice for delivering high-quality software, reducing bugs and regressions, increasing code maintainability, and building confidence in your codebase.
Unit testing
Unit testing is a software technique where individual units of code, typically functions or methods, are tested independently to ensure they behave as expected. The goal of unit testing is to isolate and validate the smallest testable components of an application to verify their correctness.
Some key characteristics of unit tests are:
- Isolation: Unit tests isolate code units, allowing for independent testing and control over dependencies.
- Independence: Each unit test is independent, avoiding interference between tests and facilitating debugging.
- Speed an efficiency: Unit tests are fast and lightweight, this enables frequent execution during development for quick feedback.
- Automation: Unit tests are automated using specialized tools and frameworks, improving efficiency and ease of execution.
- Coverage: Unit tests provide comprehensive code coverage, testing various paths and scenarios within the units.
By implementing unit testing, developers can ensure the reliability and quality of their software.
Examples
Simple example using Jest
Suppose we have a simple function called sum that adds two numbers together. The implementation of the sum function would look like this:
CODE: https://gist.github.com/MatiasDelorenzi/47478a472ee09b106cd2c8000d1a1481.js?file=myFunction.ts
Now, let’s write unit tests for the sum function using Jest:
CODE: https://gist.github.com/MatiasDelorenzi/47478a472ee09b106cd2c8000d1a1481.js?file=myFunction.unit.spec.ts
In this example, we define a test case using the it function provided by Jest. Within the test case, we set up the necessary test data (Arrange phase) by defining two variables a and b.
Then, we call the function being tested with the data (Act phase) and store the result in a variable.
When you run this unit test, Jest will execute the sum function with the provided test data and compare the actual result with the expected result. If they match, the test passes; otherwise, it fails.
But what happens in a NodeJS project, where we have more complex logic and other function/methods calls inside the unit we want to test?
NodeJS example
Lets say we have already created:
- DatabaseUtils module that handles all the requests to the database,
- CreateUserDto that defines the structure of the data transfer object for creating a new user,
And now we want to create a UserService class to handle the user creation with the condition that the user must sent an email and a password.
CODE: https://gist.github.com/MatiasDelorenzi/47478a472ee09b106cd2c8000d1a1481.js?file=user.service.ts
Now, lets write unit tests for this class:
CODE: https://gist.github.com/MatiasDelorenzi/47478a472ee09b106cd2c8000d1a1481.js?file=user.service.unit.spec.ts
Let’s break down both tests:
‘Should throw an error if email and password are not sent':
For this test, we send an invalid body and we expect the userService to fail with an specific error, this ensures that, in case the user sends incorrect information, we won’t create any record in the database.
'Should return a string if user is created':
This test introduces a super useful and interesting concept called mocking, a technique used to simulate the behavior, in this case, of the databaseUtils module. By doing this, we avoid making a real call to the database by forcing the method to return what we want since we are trying to isolate the UserService methods and test them independent of what the database might return.
So first we create a valid body, with an email and password and override the response from the database.
After that we store the service response in a variable.
Finally, we compare it with the response that we expect to receive. If they match, the test passes; otherwise, it fails.
Integration testing
Integration testing is a critical software testing technique that focuses on validating the interactions and integration between different components or modules of an application. It aims to identify issues that arise when multiple components are combined and integrated into a larger system.
Some key characteristics of integration tests are:
- Component integration: Integration testing verifies how different components, modules, or subsystems of your application work together.
- Test scenarios: Integration tests cover specific scenarios to simulate real-word usage, detecting issues related to data flow, communication errors, and compatibility conflicts.
- Dependencies: Integration testing considers the dependencies between components, ensuring correct integration of interfaces, data exchanges, and synchronization.
- Order of execution: Integration tests validate the proper sequence or order of component execution, ensuring the overall system functions correctly.
- External system and services: Integration testing involves testing interactions with external systems or services like databases, APIs, or third-party systems to validate compatibility and integration.
Incorporating integration testing into your software development process is vital for achieving a reliable and functional system.
Types of integration testing
Top-down integration testing
Top-down integration testing is a testing strategy that ensures the integration and functionality of higher-level modules before gradually integrating lower-level modules. It helps uncover issues in the system's overall flow and functionality, providing early feedback and allowing for efficient development and testing processes.
Benefits of top-down integration testing include:
- Early identification of architectural issues: By testing the main control modules first, top-down integration testing helps identify architectural or design flaws in the system's overall structure.
- Faster detection of critical issues: Top-down integration testing allows for the early detection of critical issues that could impact the system's core functionality or user experience.
- Efficient utilization of resources: The use of stubs or mock objects during testing allows developers to work on higher-level modules and functionalities while lower-level modules are still under development.
- Progressive development and testing: Top-down integration testing supports an incremental and iterative development approach, enabling the progressive refinement and integration of modules.
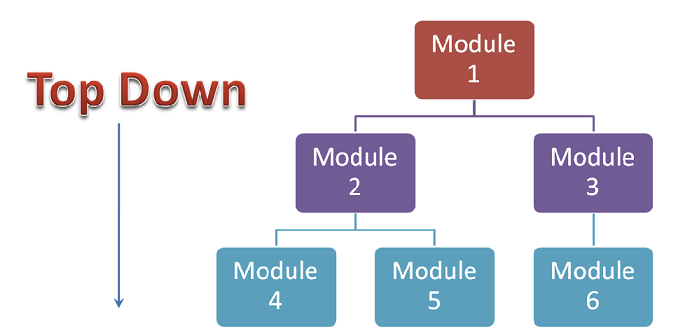
Bottom-up integration testing
Bottom-up integration testing is a testing strategy that ensures the integration and functionality of lower-level modules before gradually integrating higher-level modules. It helps uncover issues at the component level and facilitates the efficient development and testing of the system's building blocks.
Benefits of bottom-up integration testing include:
- Early identification of component-level issues: By testing the lower-level modules first, bottom-up integration testing helps identify issues or defects within the individual components of the system.
- Faster isolation of specific module issues: Bottom-up integration testing allows for the early detection and isolation of issues specific to lower-level modules, facilitating quicker resolution and debugging.
- Efficient utilization of resources: The use of drivers during testing allows developers to work on lower-level modules and functionalities while higher-level modules are still under development.
- Progressive development and testing: Bottom-up integration testing supports an incremental and iterative development approach, enabling the progressive refinement and integration of modules.
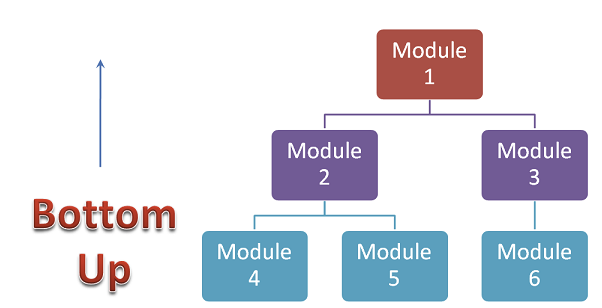
Hybrid/sandwich integration testing
Hybrid/sandwich integration testing is a hybrid approach that combines elements of top-down and bottom-up integration testing. It offers a balanced and iterative approach to integration and testing, aiming to ensure the proper integration and functionality of modules from different layers of the system.
Benefits of mixed/sandwich integration testing include:
- Balanced approach: Mixed integration testing combines the advantages of both top-down and bottom-up approaches, allowing for a balanced integration and testing process.
- Early identification of issues: By integrating and testing modules from different levels simultaneously, mixed integration testing helps identify issues or mismatches between the top and bottom layers at an earlier stage.
- Improved coverage: The simultaneous integration of modules from different levels enhances test coverage, ensuring that the interactions between different layers are thoroughly tested.
- Progressive development and testing: Mixed integration testing supports an iterative development and testing process, gradually refining the system's functionality and interactions.

Big-bang integration testing
Big-bang integration testing is an approach where all modules of a system are integrated and tested together as a complete unit. It is relatively simpler but carries higher risks compared to incremental integration testing approaches. It may be suitable for smaller systems or projects with limited module complexity and interactions.
Benefits and considerations of big-bang integration testing include:
- Simplicity and speed: Big-bang integration testing can be relatively simple and faster to execute compared to incremental integration testing approaches since it involves fewer intermediate integration steps.
- Efficient for small systems: It may be suitable for small systems or projects where the number of modules is limited, and the complexity of interactions is relatively low.
- Limited visibility and issue identification: The delayed integration approach in big-bang testing makes it challenging to identify and resolve issues early in the development process. This can lead to potential difficulties in debugging and resolving integration-related problems.
- Higher risk: Big-bang integration testing carries a higher risk since it postpones the identification and resolution of integration issues until later stages of development. This can result in larger-scale issues that may require significant effort to address.
Example
In the realm of NodeJS API development, the effectiveness of integration testing cannot be understated. As APIs consist of endpoints that facilitate communication between various components, integration testing becomes essential to validate the behavior of these endpoints. By creating an application and meticulously interacting with its endpoints using different inputs and evaluating their responses, integration testing ensures the seamless integration and optimal performance of the API.
Let’s imagine we already created an endpoint to create users in our application that must follow one of this behaviors:
- If the data is correct, it returns the new user id with a 201 HTTP status code.
- If the email or password is missing, it returns a message with this error with 422 HTTP status code.
Now, lets create tests to check that these features work as expected:
CODE: https://gist.github.com/MatiasDelorenzi/47478a472ee09b106cd2c8000d1a1481.js?file=user.integration.spec.ts