With SwiftUI, you can create attractive apps on Apple platforms, and you can do so with a small amount of code using APIs and a single set of tools.
In this article, I will help you understand how to use stack views with SwiftUI and unlock the power to build complex layouts using primitive container views.
SwiftUI Stack Views
SwiftUI has three types of primitive stack views: VStack, HStack, and ZStack, each functioning individually as simple views.
VStack refers to ‘Vertical Stack,’ HStack to ‘ Horizontal Stack,’ and ZStack to ‘Depth Stack.’
What are Stack Views?
Stacks Views are useful when we need to translate a design into code, and they are a great option for arranging static views in one dimension.
Stack Views can improve code quality dramatically by creating smaller components, breaking down complexity, and enhancing our maintenance capability.
In SwiftUI, like the VStack, container views can only contain up to 10 views. If, for some reason, you need more than 10 subviews (more than 1 view can create an error), you can use the nesting capability.
We can add a new StackView as a subview of a parent StackView; however, if you reach this point, it could be necessary to incorporate some type of refactoring into components or look for some other layout alternative.
VStack, HStack, and ZStack
VStack and HStack have alignment, content, and spacing parameters. ZStack has only alignment and content parameters.
The following table breaks down all three stacks:
Stack View Alignments
Each stack view type offers different types of alignment options. The default value for all three options is center. Stack view constructors allow us to set the different alignment options easily as follows:
CODE: https://gist.github.com/devs-rootstrap/7919545e4809b875eb28a1e41187bb96.js?file=stack-definition-example
I recommend learning about Lazy Stack Views for dynamic arranging—more on this in a future article. Let's continue with some examples of stack views.
VStack Example
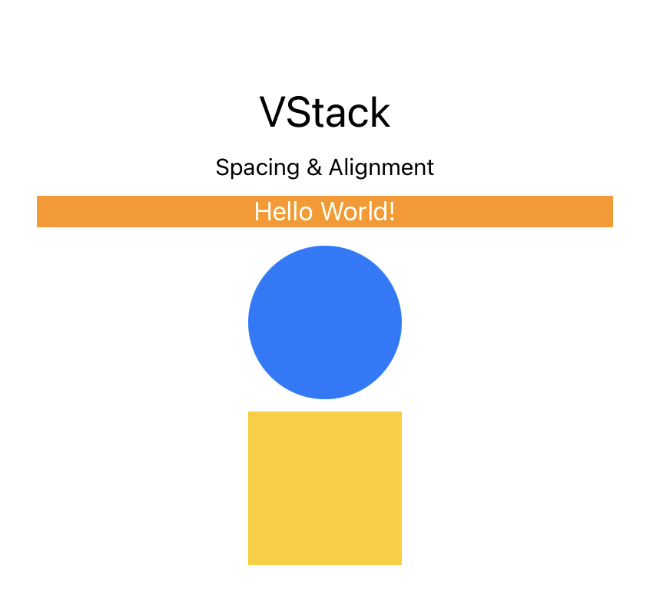
CODE: https://gist.github.com/devs-rootstrap/7919545e4809b875eb28a1e41187bb96.js?file=vstack-example
HStack Example

CODE: https://gist.github.com/devs-rootstrap/7919545e4809b875eb28a1e41187bb96.js?file=hstack-example
ZStack Example
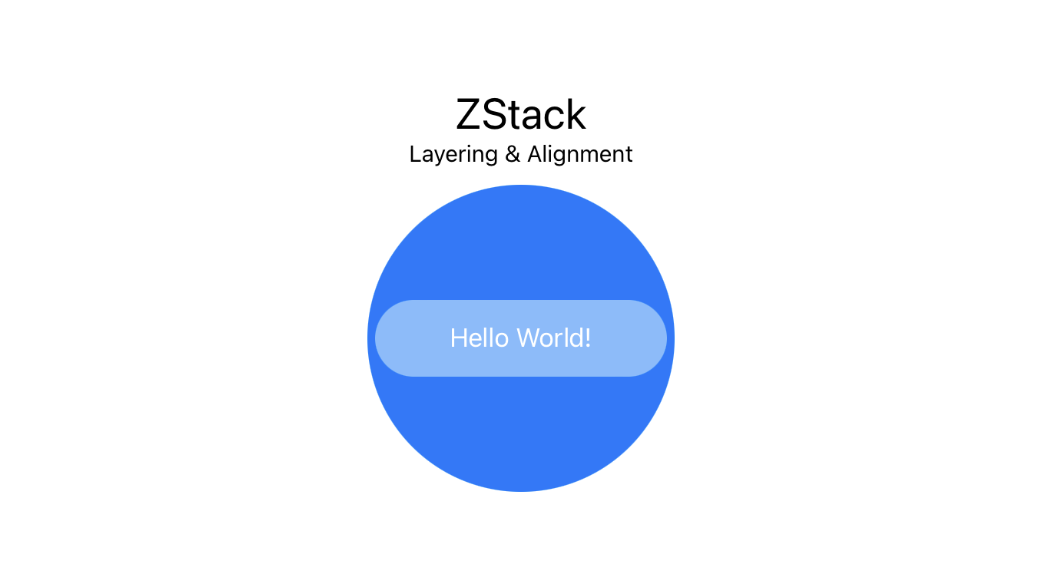
CODE: https://gist.github.com/devs-rootstrap/7919545e4809b875eb28a1e41187bb96.js?file=zstack-example
Building a Contact Detail View using Stack Views
Let's use what we learned so far to create a contact details view with some actions. Here are some things to take into account for the following example:
- We use a VStack { … } as the highest level in the view hierarchy.
- We are using StackView nesting capabilities.
- The avatar is built using a ZStack { .. }.
- We are handling some spacing through Spacer().
- Button subview is made with an HStack { … }.
- Button’s images are SF Symbols.
Contact Example
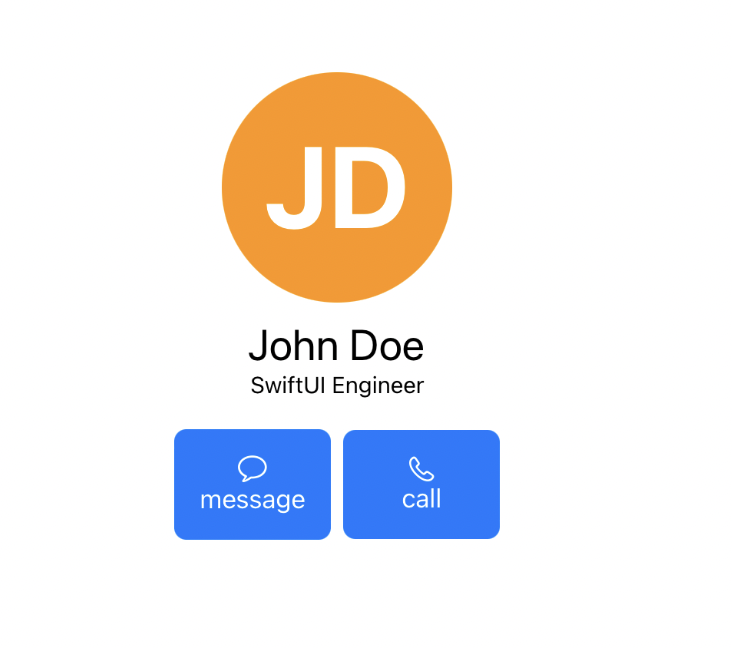
CODE: https://gist.github.com/devs-rootstrap/7919545e4809b875eb28a1e41187bb96.js?file=stack-example
The Takeaway
Stack Views must be used if we want to build complex interfaces while keeping our implementation simple and maintainable. Stack Views are a very flexible and easy way to build user interfaces.
Stack Views are part of the Layout Views provided by SwiftUI to simplify our work, and as we highlighted in this article, we can do a lot with them and convert them into a superpower.
What are your thoughts on Stack Views and Swift UI? Let us know in the comments section.