In a previous post, we talked about how to use ChatGPT with Node.js and TypeScript, now is the time to do the same with Gemini, the latest multimodal AI solution from Google.
This post shows a step-by-step guide to building a Node.js (TypeScript-based) REST API to interact with an advanced artificial intelligence language model developed by Google (Gemini).
To use Gemini with Node.js (or any other language) we need to use Gemini API. For that purpose, we can use the google generative-ai package, which provides a simple way to interact with the Gemini API and has TypeScript support.
Starting from scratch we are going to set up our project, create Gemini API keys, build our API controller, and make the API to be able to establish a historical conversation in natural language. No matter if you are a skilled developer or you just started with Node, this blog post will provide you with a clear guide to building the whole thing.
Code hands-on
Step 1: Install Node.js and TypeScript
First, you need to install Node.js on your computer if you haven't already done so. You can download and install Node.js from the official website: https://nodejs.org/en/download/
Next, you need to install TypeScript globally using the following command:
Step 2: Create a new TypeScript project
Once TypeScript is installed, you need to create a new directory for your project and navigate to it by:
Then, you can initialize a new TypeScript project by running the following command:
This will create a new tsconfig.json file in your project directory, which will contain some default information about your project and its TypeScript settings.
Step 3: Install Dependencies
Now, we need to install the dependencies for building a REST API with Node.js and TypeScript. We can do this by running the following command:
This will install the following packages:
- express: a popular web framework for Node.js
- body-parser: middleware for parsing request bodies
- @google/generative-ai: a package for accessing the Gemini model
- dotenv: loads environment variables from a .env file
- @types/node: TypeScript type definitions for Node.js
- @types/express: TypeScript type definitions for express
- @types/body-parser: TypeScript type definitions for body-parser
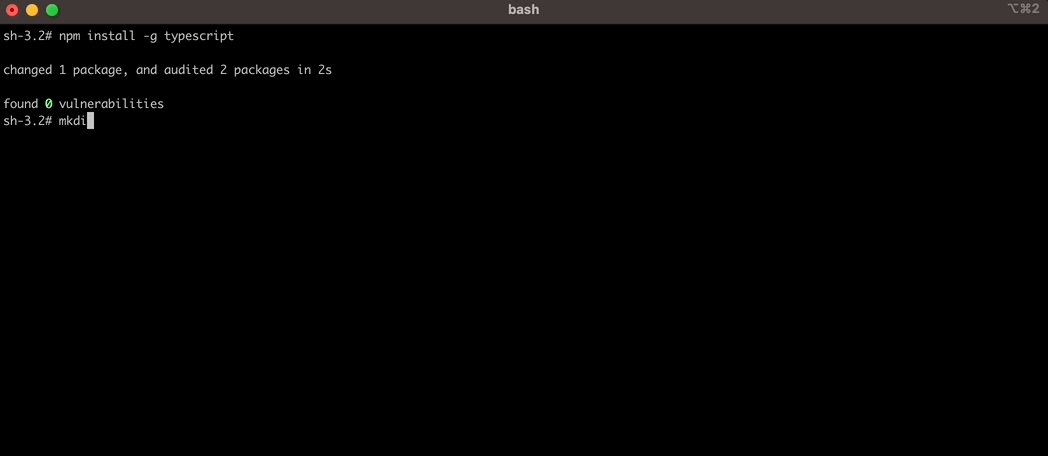
Step 4: Get Up Gemini API Credentials
Before using Gemini, you need to set up API credentials for the API. To do this, you need to sign up on your Google account and create an API key. You can do this here: https://makersuite.google.com/app/apikey
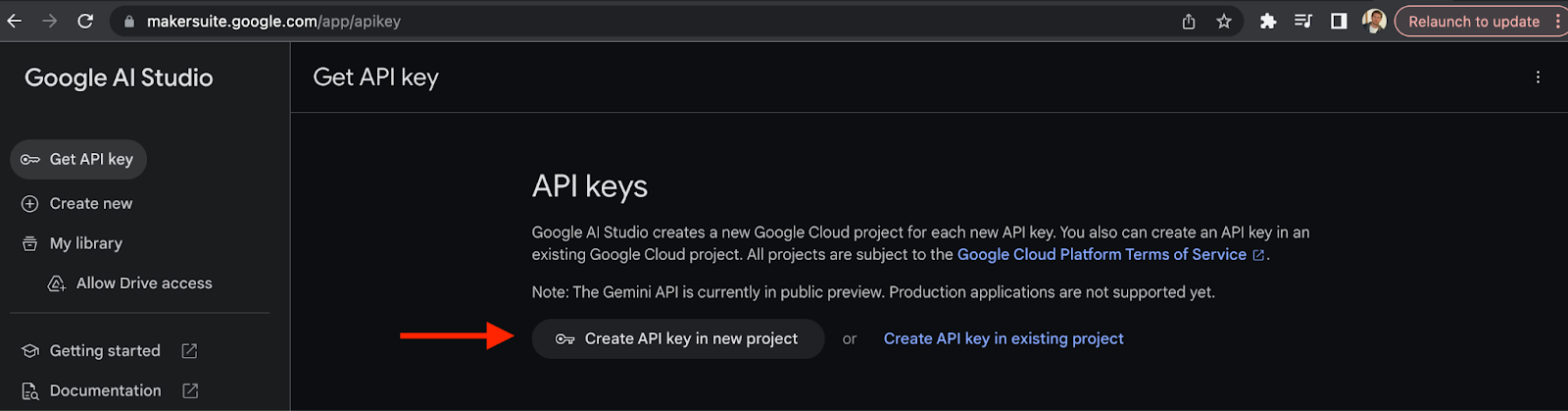
Once you have your API key, create a .env file in your project directory and place these variables:
Step 5: Create an Express server and Gemini interaction
Now, you can create an Express server to handle HTTP requests and responses. Create a new TypeScript file called app.ts in your project root directory, and add the following code:
This code sets up an Express server with body-parser middleware. It also loads environment variables from .env file by using dotenv library.
Next, we must create a new controller function to handle the generateResponse route declared in the above code. Create a new folder called controllers in the root directory of the project and inside of that folder a new file called index.ts , and add the following code to it:
In this example, we are using the gemini model which can take the history of the conversation into account, and you can ask subsequent questions based on the context of the conversation that has taken place in a previous prompt.
Step 6: Build and Run
Build your application and start the server using the following commands:
This will compile the typescript code to javascript and start the server on port 3000.
Step 7: Test the API using a client
You can test the REST API using any HTTP client like Postman or PostCode. Send a POST request to http://localhost:3000/generate with the following JSON payload:
That will generate a response using Gemini and return it in the response body:
Given that we implemented the controller to be able to use the history of the conversation, we can make another question to Gemini based on the previous one.
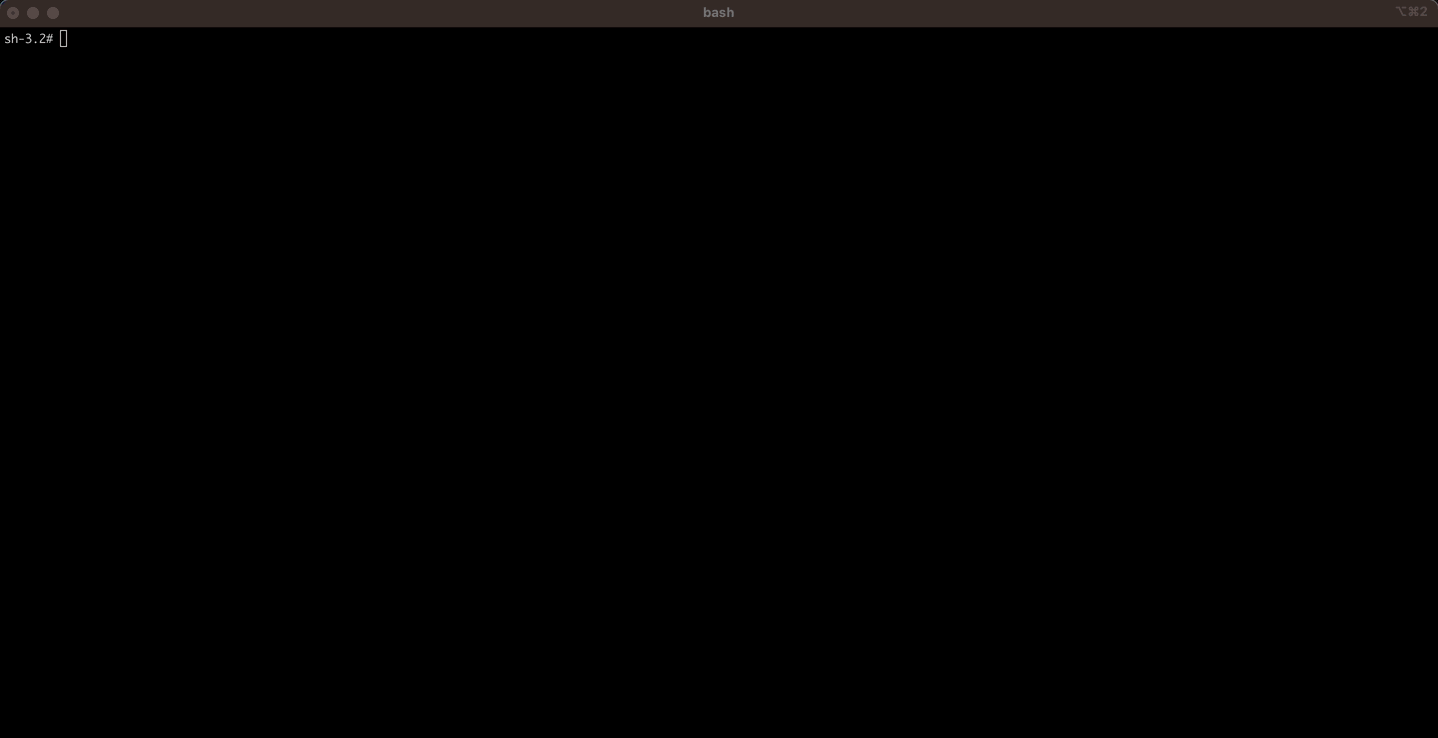
That's it! You have now successfully built a REST API with Node.js and TypeScript to interact with Gemini.
Code repository example
You can get the working code example from this repository: https://github.com/rootstrap/gemini-nodejs
Summary
With this basic guide, you are now able to interact with Gemini through your custom Node.js API and use it as a base for your projects. There are several ways you can extend this REST API we just built, to make it more useful and robust by adding some new features like more routes to interact with Gemini API, create input validation, error handling, authentication, and caching, among others.
These are just a few ideas where you can get started. There are many other ways you can extend the API depending on your specific requirements and use cases. The key is to start small and gradually add new features as needed.
Also, remember that Gemini offers other models than gemini-pro like gemini-pro-vision or embedding-001, you can learn more about that here: https://ai.google.dev/models/gemini