View Modifiers in SwiftUI are functions or methods used to modify the appearance or behavior of views in a declarative and reusable manner. They enable developers to apply consistent styles, layouts, and interactions to their user interface components, enhancing code readability, organization, and maintainability.
Essentially, view modifiers allow developers to encapsulate view modifications and apply them to multiple views throughout their SwiftUI application, promoting code reusability and efficient development workflows. In this article, we'll explore the purpose and benefits of View Modifiers and how they enhance the development experience.
In SwiftUI, view modifiers are used extensively to customize the appearance and behavior of views. Here are some common examples of view modifiers and how they're used:
Examples of View Modifiers in SwiftUI
Modifying Text Appearance
CODE: https://gist.github.com/JUNIOR140889/32c70f3e38eecb27b5a9f442f1511841.js?file=textAppearence.swift
Adding Borders and Corners
CODE: https://gist.github.com/JUNIOR140889/2f52b4f313dfd91f81b93ba1393f75e1.js?file=borderAndCorners.swift
Applying Shadows
CODE: https://gist.github.com/JUNIOR140889/e5ac2f52398c2d583194122aa275e186.js?file=applyingShadow.swift
Frame Adjustments
CODE: https://gist.github.com/JUNIOR140889/f3d712d7b6d55a8ac44a77a5707653cf.js?file=frameAdjustments.swift
Creating Custom View Modifiers
You can also create your own custom view modifiers for repetitive styling. This will be covered in greater detail subsequently.
Advantages of View Modifiers in SwiftUI
SwiftUI's View Modifiers play a crucial role in the framework, offering a declarative approach to designing and customizing user interfaces. They encapsulate the modification of views in a reusable, concise, and readable manner. Here are some key advantages of using View Modifiers in SwiftUI:
Encapsulation and Reusability
View Modifiers allow developers to encapsulate a set of view modifications into a single, reusable entity. This means that you can define a specific set of styling rules or behavior modifications once, and then apply them to multiple views throughout your application. This encapsulation promotes reusability, improves code organization and reduces potential bugs.
Consistency in Design
Maintaining a consistent look and feel across an application is crucial for providing a seamless user experience because it creates a consistent and familiar interface, leading to easier navigation, trust in the product, and strong brand recognition. By creating View Modifiers for common design elements like fonts, colors, spacing, and more, developers can enforce a unified design language.
Readability and Organization
ViewModifiers enhance code readability by isolating view modifications from the main body of the view. Instead of cluttering the view hierarchy with inline styling or behavior code, developers can apply a ViewModifier to convey the specific intent behind the modification. This separation of concerns makes the codebase cleaner, easier to understand, and more maintainable.
Easy Maintenance
When a global change to the styling or behavior of views is required, ViewModifiers shine. By updating the ViewModifier, the changes automatically propagate to all views that utilize it. This reduces the potential for inconsistencies in the app's design and streamlines the maintenance process.
Composition
ViewModifiers can be composed together, allowing developers to combine different styles or behaviors as needed. This enables the creation of complex UI elements by assembling simpler modifiers. The ability to stack and reuse modifiers facilitates the construction of intricate interfaces without sacrificing readability or maintainability.
Custom SwiftUI ViewModifier example
So let's consider a real-life example where we will need to use a custom ViewModifier in SwiftUI.
Scenario: Suppose you're developing a weather app where you frequently display temperature readings throughout the application. You want to apply consistent styling to these temperature views to maintain a cohesive look and feel.
Solution: You can create a TemperatureStyle ViewModifier to encapsulate the styling for temperature views.
CODE: https://gist.github.com/JUNIOR140889/f398624fac45653010827113e674d791.js?file=temperatureStyle.swift
To create a custom ViewModifier, you define a struct that conforms to the ViewModifier protocol. This protocol requires you to implement the `body(content:)` method, where you specify how the view should be modified.
Accessing the view modifier through a view extension
You can access a ViewModifier through a view extension in SwiftUI by creating a function that applies the modifier. This allows you to use a more convenient syntax when applying the modifier to a view.
CODE: https://gist.github.com/JUNIOR140889/09607410b91248e11dded184ccfc2673.js?file=temperatureModifier.swift
Now, whenever you want to display a temperature reading, you can apply this TemperatureStyle modifier to ensure consistent styling:
CODE: https://gist.github.com/JUNIOR140889/7d556317bd205e3290a0c72d4278fd8a.js?file=temperatureStyleExample.swift
This will apply the specified font, color, padding, background, and corner radius to the temperature text, ensuring a uniform appearance throughout your app.
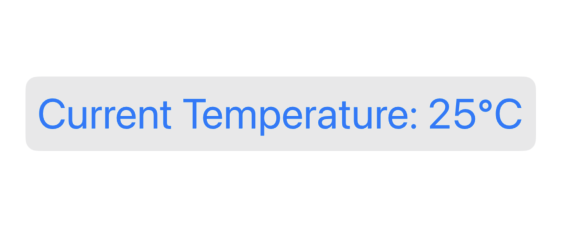
It is important to mention that in SwiftUI the order of applying ViewModifiers can influence the final appearance of the view. When you apply multiple modifiers to a view, SwiftUI processes them in the order they are declared. This means that modifiers applied later can override or modify the effects of modifiers applied earlier.
Let's modify the order of the View Modifiers to see how it changes the final result. If another green background is added above the padding modifier, then the color is applied only to the text, not including the padding.
CODE: https://gist.github.com/JUNIOR140889/6c37f6ffb5b6553d92b88034c46f6c5d.js?file=temperatureStyleOrder1.swift

In case the green background modifier above the padding is the only one, you will not be able to see the corner radius, as it will be applied after the padding, which has no color, so it seems that the corner radius has no effect.
CODE: https://gist.github.com/JUNIOR140889/5e516bb280caebbf02e21ffb682db845.js?file=temperatureStyleOrder2.swift
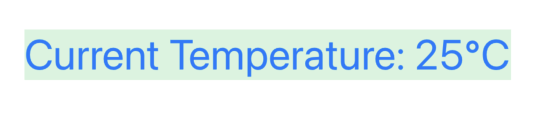
Summary
When working with SwiftUI, it's crucial to think about the final look and feel you want to achieve, and ensure that view modifiers are applied in the correct order. ViewModifiers represent a powerful feature of SwiftUI, enhancing code reusability, maintainability, and design consistency. By encapsulating view modifications, developers can create cleaner, more organized code, leading to more efficient development workflows and ultimately, a better user experience. Incorporating ViewModifiers into your SwiftUI projects can greatly enhance your ability to create elegant and functional user interfaces.