Vuelidate is an adaptable and user-friendly form validation solution designed for Vue.js. In this tutorial, I will walk you through the process of installing and configuring Vuelidate within a Vite + Vue project.
Step 1: Setting Up a Vite + Vue Project
To begin, let's initiate a new project using Vite. We'll utilize "create-vite," a tool that enables rapid generation of project templates for popular frameworks:
CODE: https://gist.github.com/highmax/27fa0faaa3c0e990e48baad5fa130c9e.js?file=createVite.bash
Once the project is generated, navigate to its directory:
CODE: https://gist.github.com/highmax/27fa0faaa3c0e990e48baad5fa130c9e.js?file=changeDirectory.bash
Step 2: Installing Vuelidate
Vuelidate 2.x is compatible with Vue 3. To install Vuelidate, we need to include the following dependencies:
CODE: https://gist.github.com/highmax/27fa0faaa3c0e990e48baad5fa130c9e.js?file=installVuelidate.bash
Step 3: Configuring Vuelidate for Form Validation
In earlier versions of Vuelidate (0.x), we could apply it globally across our application using the Vuelidate plugin. However, this approach has been removed in the latest versions. The current approach involves using the useValidate function within the component we intend to validate.
Let's proceed by creating a basic registration form with fields for name, email, password, and password confirmation. We'll place this form in a new component named ‘RegisterForm.vue’ within the components folder.
CODE: https://gist.github.com/highmax/27fa0faaa3c0e990e48baad5fa130c9e.js?file=RegisterForm.vue
The fields are validated based on the rules defined in the "rules" object. If any field fails the validations, an error message is displayed associated with the corresponding field. When the form is submitted, the "handleSubmit" function validates the fields using Vuelidate and shows an alert with the result of the form submission.
Code Breakdown
Importing Modules:
CODE: https://gist.github.com/highmax/27fa0faaa3c0e990e48baad5fa130c9e.js?file=imports.js
The previous code snippet begins by importing essential functionalities from both Vue and Vuelidate. It employs the reactive and computed elements to enable dynamic updates and efficient computations. The code further utilizes the useVuelidate function from the Vuelidate library. Additionally importing some validators that we will use later in our validation rules.
Reactive Form Object:
CODE: https://gist.github.com/highmax/27fa0faaa3c0e990e48baad5fa130c9e.js?file=reactiveForm.js
Creating a form object using Vue's reactive function, serving as a container for the values of form fields. This object is initially populated with empty strings, and as users engage with the form on the user interface and input data, the content of these fields within the object dynamically updates to reflect the entered values.
Validation Rules:
CODE: https://gist.github.com/highmax/27fa0faaa3c0e990e48baad5fa130c9e.js?file=computedRules.js
We created a rules object which will serve as validation guidelines for each form field. These rules are wrapped within a function that keeps them responsive, allowing them to update when related fields change. In this order, specific requirements are defined like this: the name field must have a value, the email field should contain a valid email address, the password field must be at least 8 characters long, and the confirmPassword field needs to match the value entered in the password field.
Step 4: Testing the Form
Integrate the form into your main component (App.vue) for testing purposes:
CODE: https://gist.github.com/highmax/27fa0faaa3c0e990e48baad5fa130c9e.js?file=App.vue
Now, you can launch the Vite development server to test the form and its validations:
CODE: https://gist.github.com/highmax/27fa0faaa3c0e990e48baad5fa130c9e.js?file=runProject.bash
Empty Fields & Error Messages
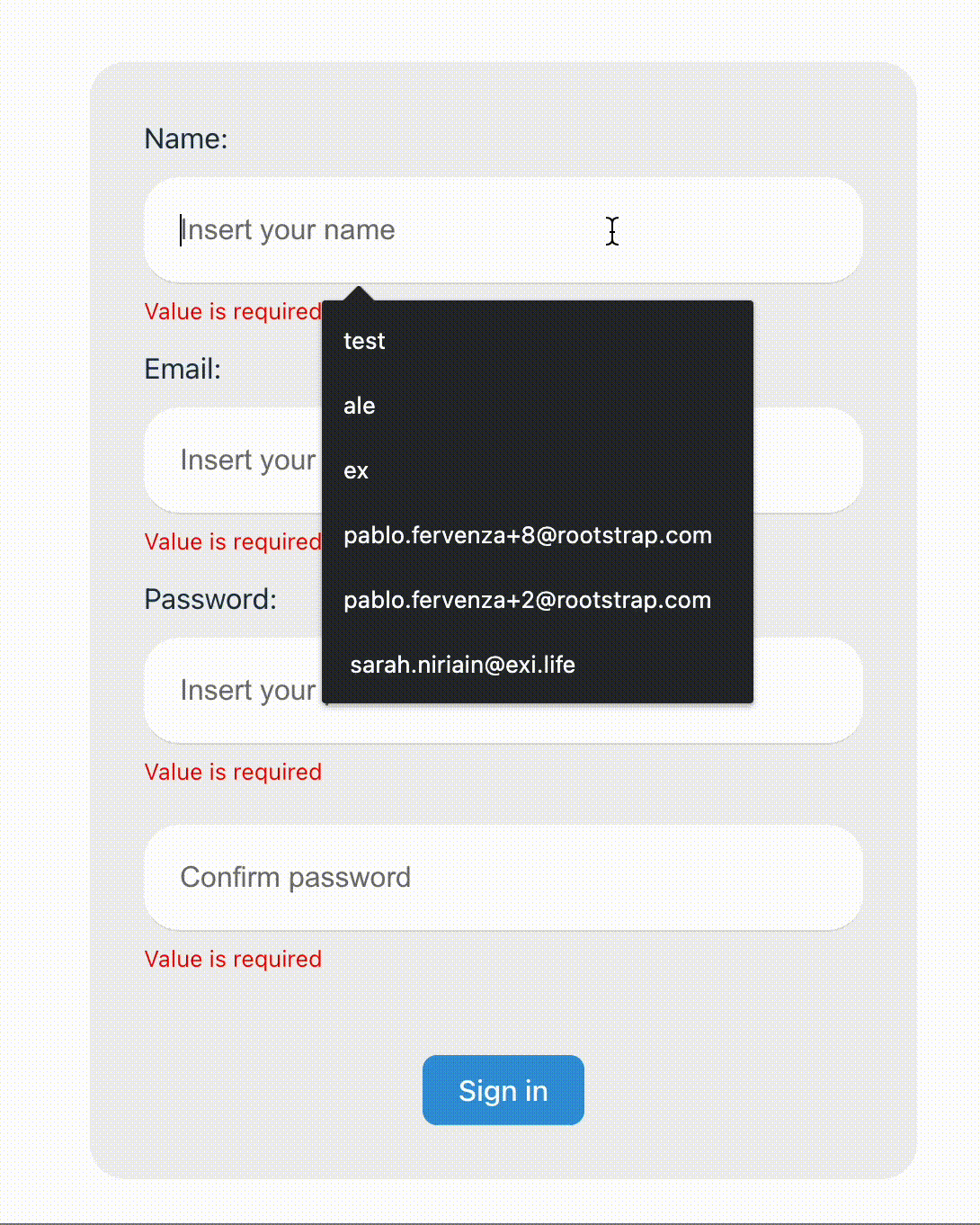
Password Validation
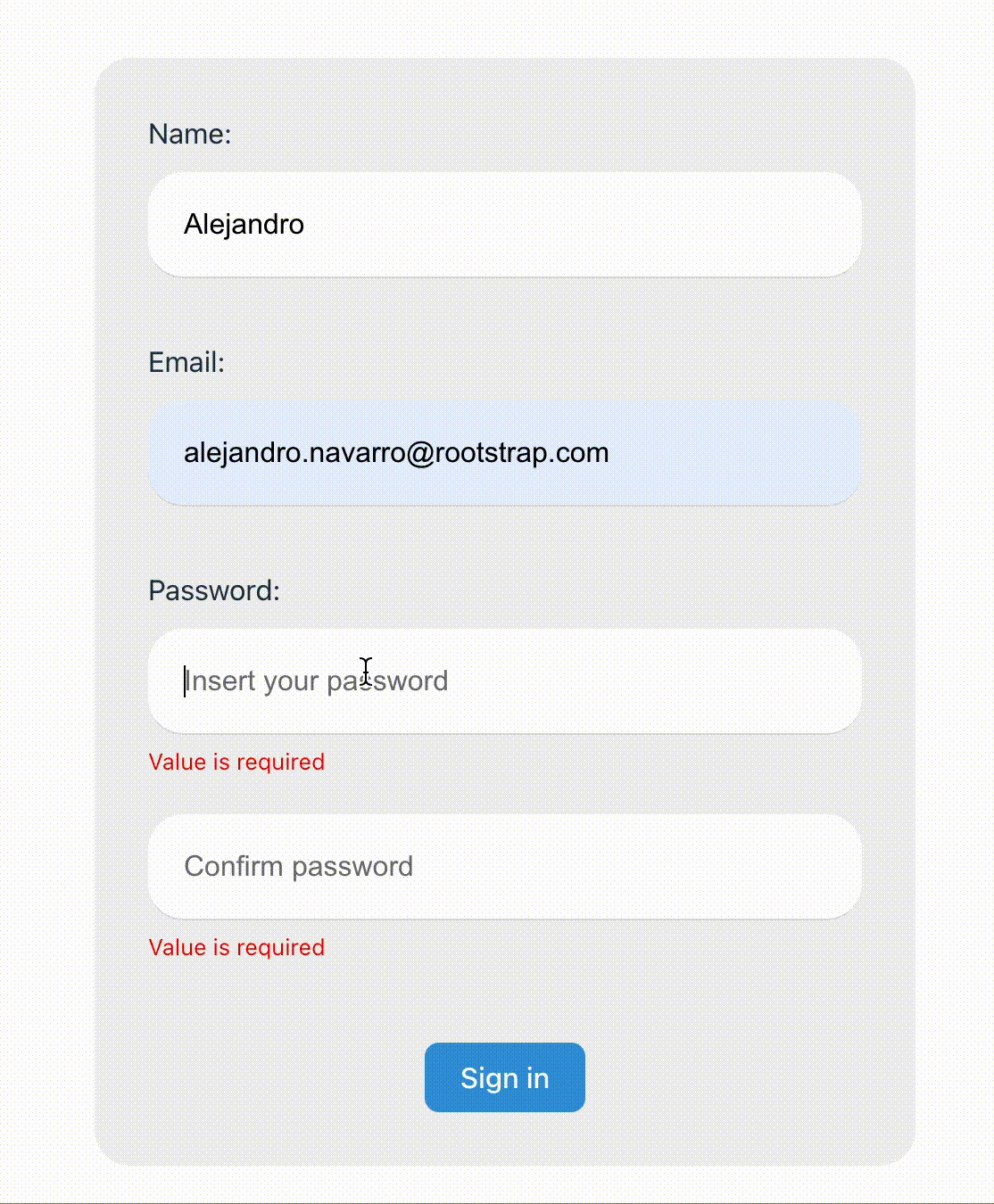
Successful Validation
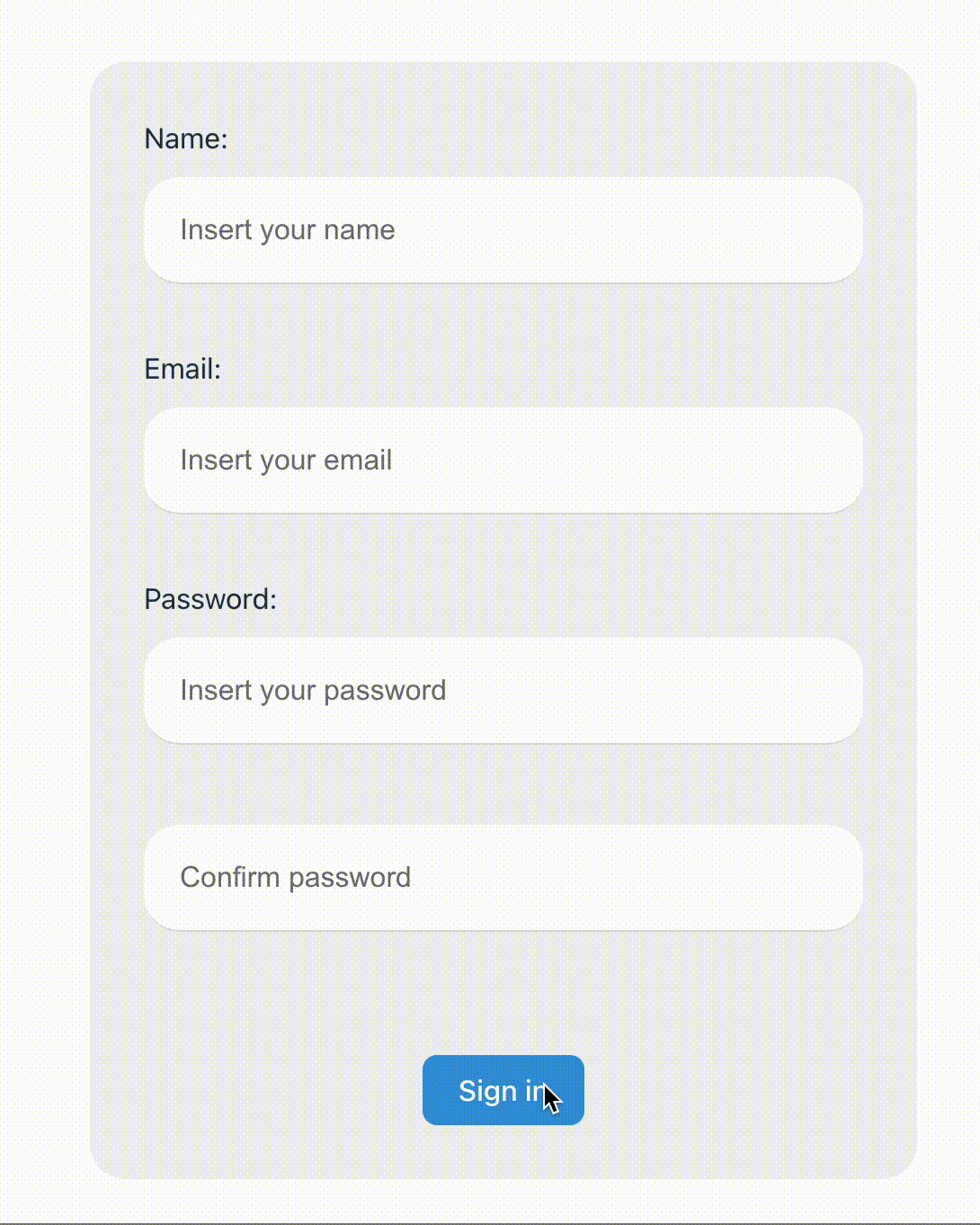
Step 5: Conclusion and next steps
With this setup, you can effectively assess form validation using Vuelidate within your Vite + Vue project. Remember that Vuelidate offers a variety of validators beyond those covered here. For a complete list of validators, refer to the official documentation. If you're up for a challenge, you can even create your own custom validators.
Happy coding!