We have previously discussed Django REST framework and dj-rest-auth, both effective libraries for Django where you can easily create rest APIs and handle user authentication.
In this post, we will focus particularly on dj-rest-auth and its built-in reset password functionality. If users want to reset their password they must submit a reset password request, and receive an email with a link to a reset page.
This feature is provided by the dj-rest-auth library but the email sent to its users is in plain text, while the link that comes in it is from a built-in dj-rest-auth endpoint. This may be exactly what you want in some cases, but it’s not always a good fit.
If you want to change this email content, the process is well documented but only if you want to change it to a plain text file. If you want to change to a custom HTML template, there is not much information on this.
Set-up
For this instance, we will assume that you have python, Django, Django REST framework, and dj-rest-auth installed for a project ready to make these changes. In my example, I made a very simple project with just one app called API, and the following layout:

As you can see above, I have a templates folder in which I made a new folder called [.c-inline-code]registration[.c-inline-code] with a file called [.c-inline-code]custom_reset_confirm.html[.c-inline-code] within.
The context in the HTML email template looks like this:

As you may have noticed, the content is pretty much the same as in the default email, and for the sake of simplicity, I didn’t add a lot of styles, just some bold and italic text as well as a greeting in bigger font size.
The relevant variables like [.c-inline-code]site_name[.c-inline-code], [.c-inline-code]domain[.c-inline-code], and such, will be automatically assigned values passed on the context to the template.
Changing the default email
Now that we have our template, what we need to do next is to indicate that this is what we want to send our users when they request a password reset.
To do this, we have to define a serializer that inherits from dj-rest-auth’s [.c-inline-code]PasswordResetSerializer[.c-inline-code] and overwrite its [.c-inline-code]get_email_options[.c-inline-code] method, and then use that serializer instead of the default one.
So, first, we will define the new serializer:


And then in our [.c-inline-code]settings.py[.c-inline-code], we will indicate that this is the serializer we want to use:
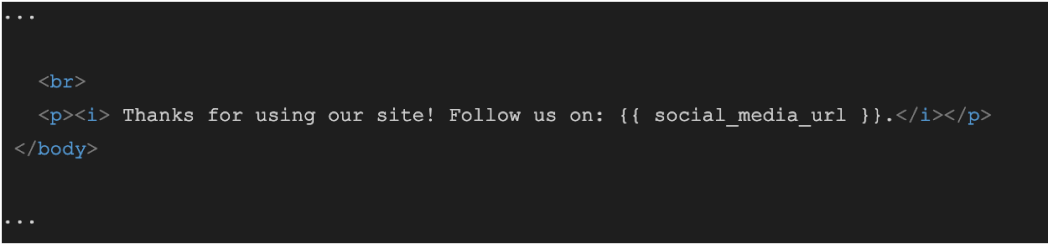
Now, if you send a reset password request you should receive something like this:
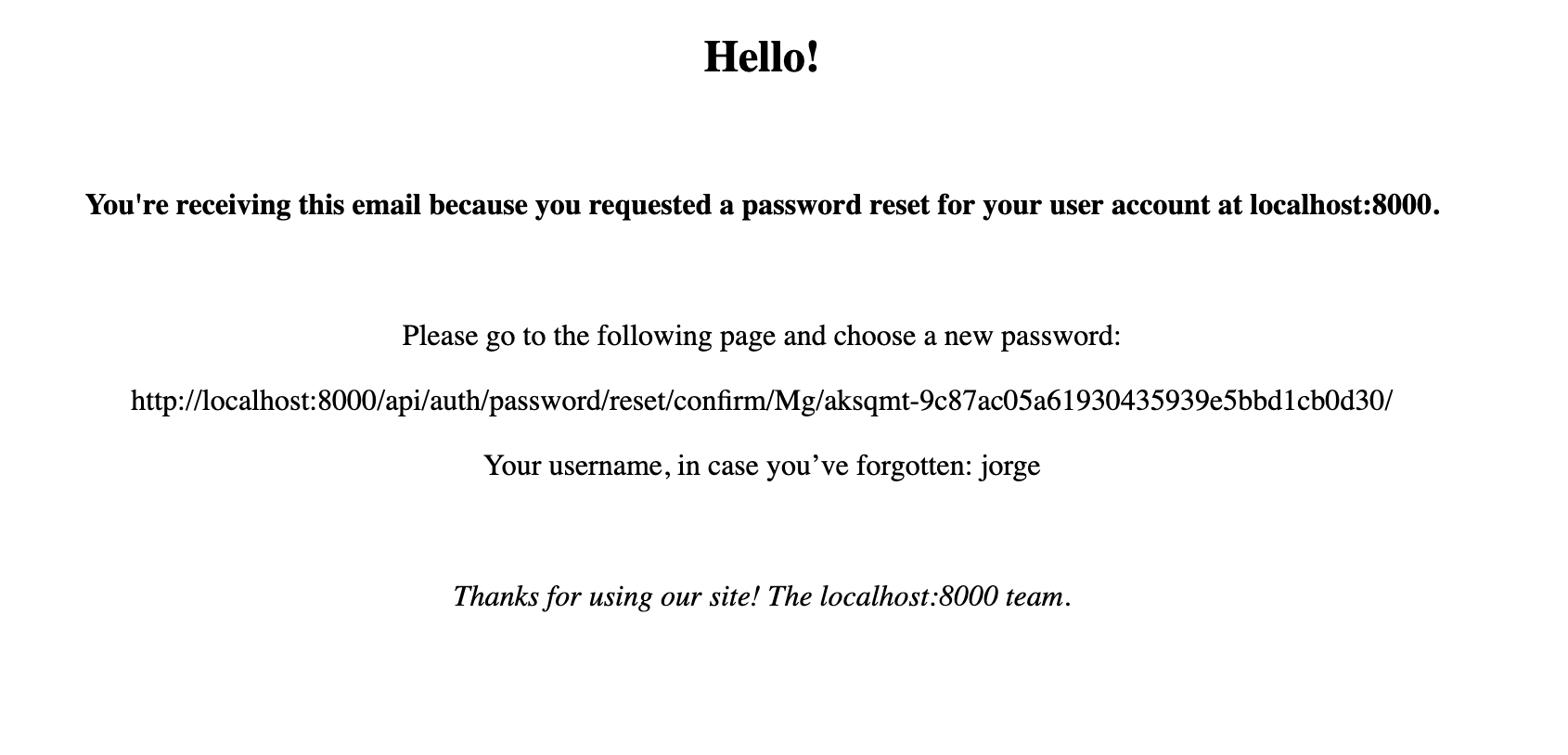
In my case, because I’m running this project locally, it uses [.c-inline-code]localhost:8000[.c-inline-code] for the [.c-inline-code]site_name[.c-inline-code].
Now, let’s say that you want to add something else to this email, some information that may change and you wouldn’t be able to hardcode it on the template itself.
Let’s take adding a link to social media, in that case, I can add the key [.c-inline-code]extra_email_context[.c-inline-code] to the dictionary that [.c-inline-code]get_email_options[.c-inline-code] returns, and as its value a dictionary with the extra context.
For example, let’s replace [.c-inline-code]The {{ site_name }} team[.c-inline-code] at the end with a fake URL:
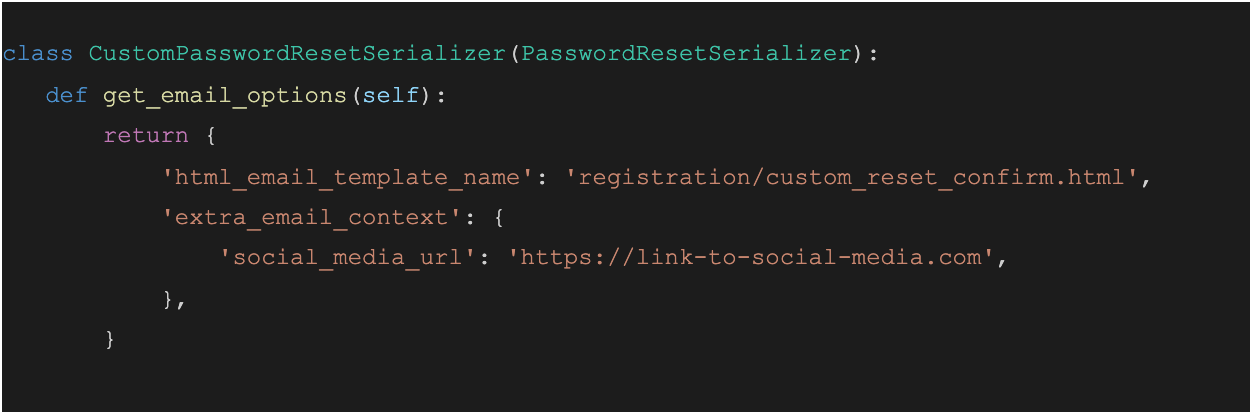
And now define the value of [.c-inline-code]social_media_url[.c-inline-code] in the serializer.
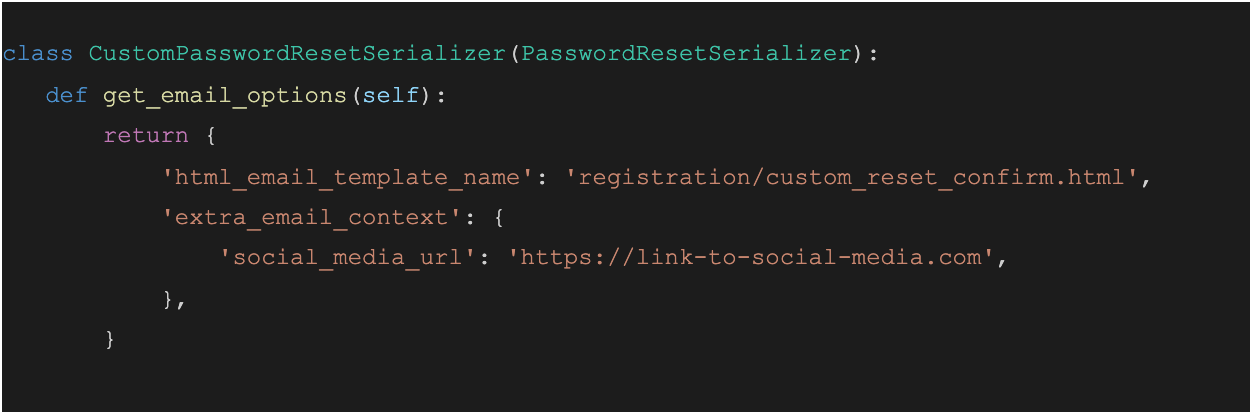
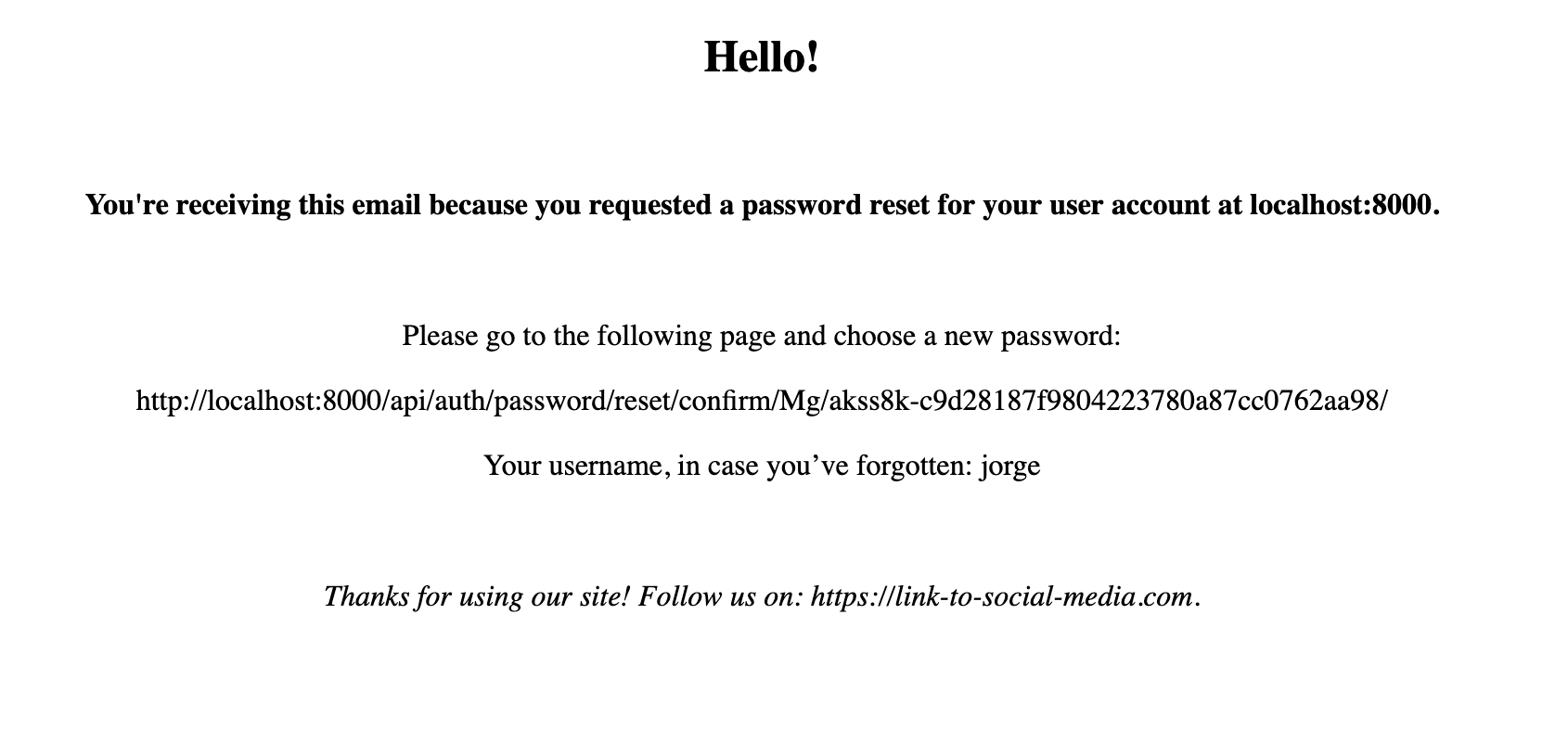
It is also possible to overwrite default values like [.c-inline-code]site_name[.c-inline-code] in the same way by using [.c-inline-code]extra_email_context[.c-inline-code], but it is not recommended to do so.
Finally, here is some code to make a simple but much prettier template so you can appreciate how much more visually appealing your email can be:
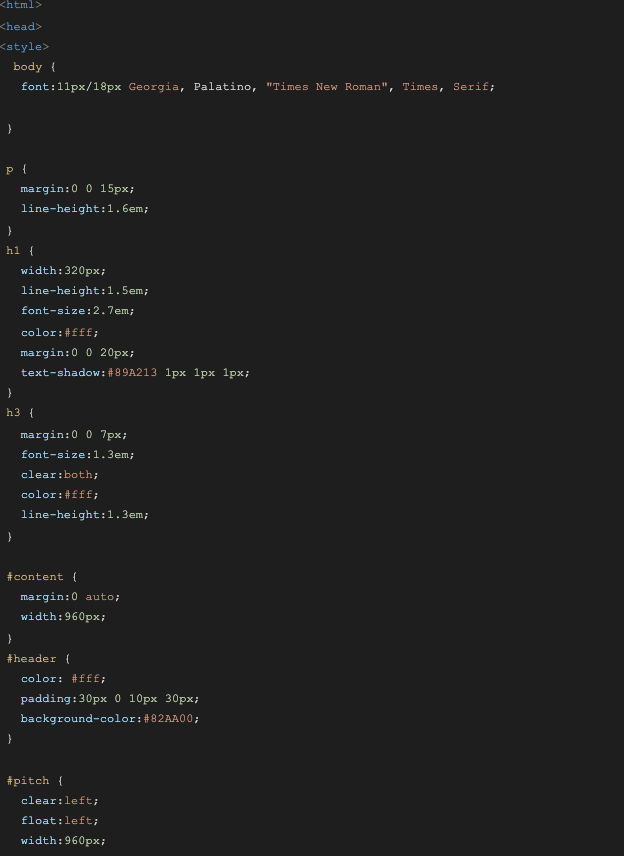
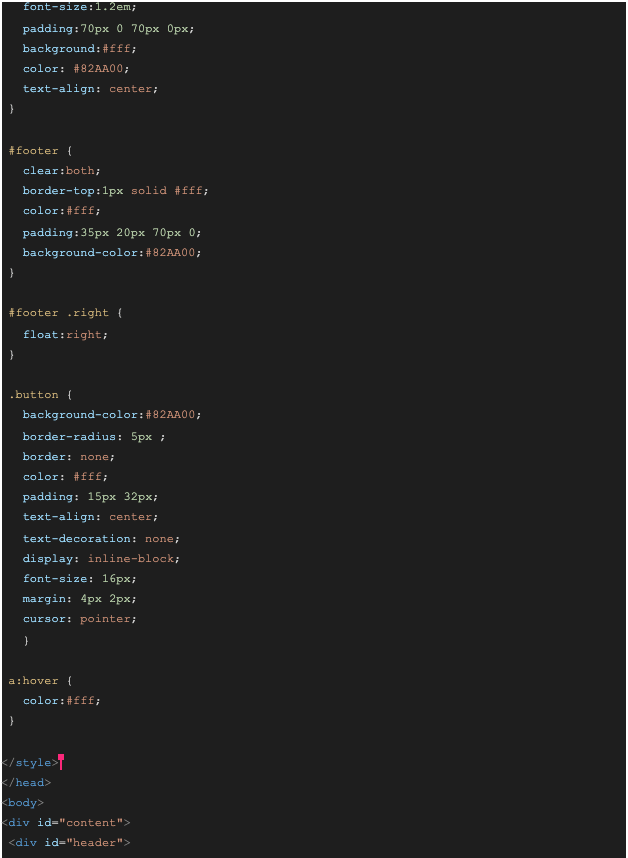
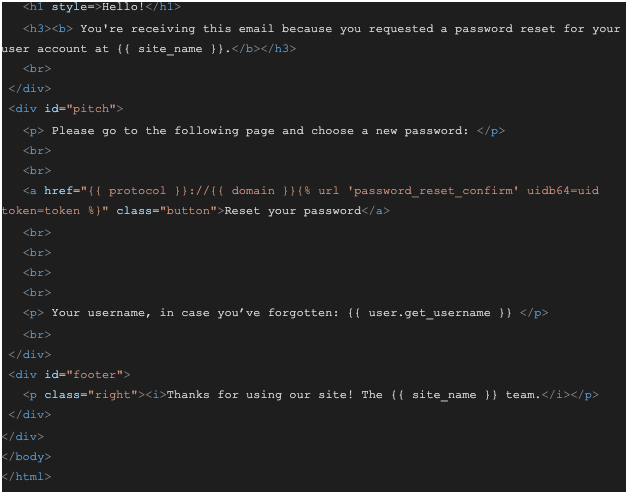
And now, this is how it will look:

Conclusion
In this blog post, you learned how to overwrite any email template in dj-rest-auth. While we focused on the reset password feature, the same principle can be used in other cases.
We replaced the reset password email with a new HTML one by defining a custom [.c-inline-code]PasswordResetSerializer[.c-inline-code]. We also updated the email content by dynamically using the get_email_options method of the [.c-inline-code]PasswordResetSerializer[.c-inline-code] base class.
I hope you enjoyed this latest post and found it useful. Thank you for reading and stay tuned for more similar content.